How to Build Instagram-Style Splash Screens with Expo 52
With the release of Expo 52, the expo-splash-screen module has been updated. In this article, I’ll cover the changes and show you how to…
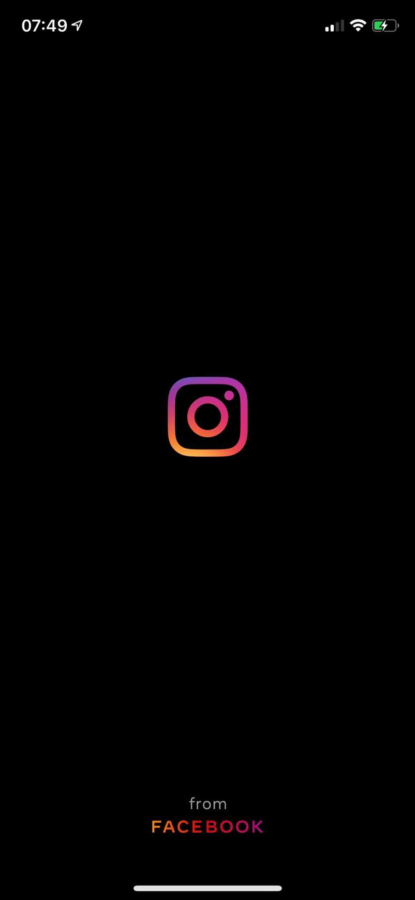
With the release of Expo 52, the expo-splash-screen
module has been updated. In this article, I’ll cover the changes and show you how to set up a splash screen like the ones in major apps such as Instagram and Facebook.
If you examine most popular apps, their splash screens tend to follow a common pattern. They typically feature an icon in the center with a background that’s black, white, or the app’s primary color. Android recently updated its splash screen API and no longer supports full-screen splash images. This approach aligns with how apps like Instagram and Facebook design their splash screens. On iOS, full-screen splash screens are still supported for now, but this feature will eventually be deprecated.
Install expo-splash-screen
npx expo install expo-splash-screen
Example App
Here is a sample implementation:
import { useCallback, useEffect, useState } from 'react';
import { Text, View } from 'react-native';
import Entypo from '@expo/vector-icons/Entypo';
import * as SplashScreen from 'expo-splash-screen';
import * as Font from 'expo-font';
// Keep the splash screen visible while we fetch resources
SplashScreen.preventAutoHideAsync();
// Set the animation options (optional)
SplashScreen.setOptions({
duration: 1000,
fade: true,
});
export default function App() {
const [appIsReady, setAppIsReady] = useState(false);
useEffect(() => {
async function prepare() {
try {
// Preload fonts or make necessary API calls
await Font.loadAsync(Entypo.font);
// Simulate a delay for demonstration purposes (remove this in production)
await new Promise(resolve => setTimeout(resolve, 2000));
} catch (e) {
console.warn(e);
} finally {
setAppIsReady(true);
}
}
prepare();
}, []);
const onLayoutRootView = useCallback(() => {
if (appIsReady) {
SplashScreen.hide();
}
}, [appIsReady]);
if (!appIsReady) {
return null;
}
return (
<View
style={{ flex: 1, alignItems: 'center', justifyContent: 'center' }}
onLayout={onLayoutRootView}>
<Text>SplashScreen Demo! 👋</Text>
<Entypo name="rocket" size={30} />
</View>
);
}
In this code:
- We prevent the splash screen from automatically dismissing using
SplashScreen.preventAutoHideAsync()
. - A fade animation is set with a default duration of 400ms (customizable).
- The app hides the splash screen once resources are ready.
Splash Screen Configuration
To configure your splash screen, modify the app.json
or app.config.js
file as follows:
{
"expo": {
"plugins": [
[
"expo-splash-screen",
{
"backgroundColor": "#232323",
"image": "./assets/splash-icon.png",
"dark": {
"image": "./assets/splash-icon-dark.png",
"backgroundColor": "#000000"
},
"imageWidth": 200
}
]
]
}
}
Key Features:
- Fade Animation: You can enable fade animations for smoother transitions.
- Light and Dark Mode: Define separate splash screen assets for light and dark themes(This is optional).
- Image Width: The default image width is 100, but you can increase it to 200 (not recommended to exceed 200). I have found that right around 100 looks good!
- Legacy Full-Screen Image Support: Use the
enableFullScreenImage_legacy
property to enable full-screen splash images on iOS only. For platform-specific configurations, includeios
andandroid
objects in your configuration.
Testing Your Splash Screen
To test the splash screen, you need to use Development Builds. Note that the splash screen in Expo Go will only display the app icon. While the splash icon might not always appear in development builds, it works as expected in TestFlight and Google Play Beta builds.
By following these steps, you can create a sleek splash screen for your Expo app that enhances your app’s user experience.
Try BanKan Board — The Project Management App Made for Developers, by Developers
If you’re tired of complicated project management tools with story points, sprints, and endless processes, BanKan Board is here to simplify your workflow. Built with developers in mind, BanKan Board lets you manage your projects without the clutter.
Key Features:
- No complicated processes: Focus on what matters without the overhead of traditional project management systems.
- Claude AI Assistant: Get smart assistance to streamline your tasks and improve productivity.
- Free to Use: Start using it without any upfront cost.
- Premium Features: Upgrade to unlock advanced functionality tailored to your team’s needs.
Whether you’re building a side project, managing a team, or collaborating on open-source software, BanKan Board is designed to make your life easier. Try it today!