Expo Router Type-Safety
If you have ever used React Navigation, you may have set up type safety for your screens. This straightforward integration likely saved you…
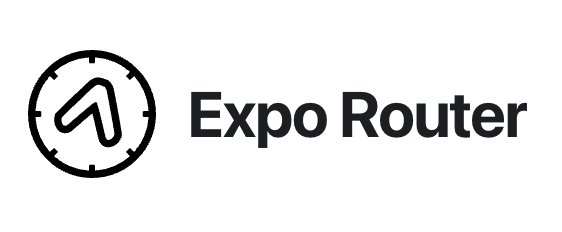
If you have ever used React Navigation, you may have set up type safety for your screens. This straightforward integration likely saved you from numerous navigation bugs. In a recent article, I explained why I decided to switch to Expo Router. Today, I will show you how to implement type safety with Expo Router.
Setting Up Expo Router
Step 1: Install Dependencies
Run the following command to install Expo Router and related packages:
npx expo install expo-router react-native-safe-area-context react-native-screens expo-linking expo-constants expo-status-bar
Step 2: Configure Your Entry Point
Add the following to your package.json
to define the entry point for Expo Router:
{
"main": "expo-router/entry"
}
Step 3: Add Deep Linking
In your app configuration (e.g., app.json
or app.config.js
), specify a custom deep linking scheme:
{
"scheme": "your-app-scheme"
}
Step 4: Update Babel Configuration
Modify your babel.config.js
file:
module.exports = function (api) {
api.cache(true);
return {
presets: ['babel-preset-expo'],
};
};
With the configuration complete, let’s dive into implementing type safety with Expo Router.
Adding Type Safety to Expo Router
Enable Typed Routes
Add the following configuration to your app.json
or app.config.js
:
{
"expo": {
"experiments": {
"typedRoutes": true
}
}
}
Generate TypeScript Configuration
Run the following command to update your tsconfig.json
for type safety support:
npx expo customize tsconfig.json
Working with Routes
Static Routes
Examples of type-safe static routes:
<Link href="/about" />
<Link href="/user/1" />
<Link href={`/user/${id}`} />
<Link href={"/user" + id as Href} />
Dynamic Routes
Examples of type-safe dynamic routes:
<Link href={{ pathname: "/user/[id]", params: { id: 1 } }} />
Using the Router
Type safety also applies when navigating with the router function:
import { router } from 'expo-router';
router.push('/about');
Working with Route Parameters
Defining Route Params
There are two ways to set up route parameter types:
import { Text } from 'react-native';
import { useLocalSearchParams } from 'expo-router';
export default function Page() {
// Option 1 - Search: string[]
const { search } = useLocalSearchParams<'app/(search)/[...search].tsx'>();
// Option 2 - Search: string[]
const { search } = useLocalSearchParams<{ search: string[] }>();
return (
<>
<Text>Search: {search.join(',')}</Text>
</>
);
}
Option 1 is preferred for most use cases as it provides stronger type inference. However, Option 2 will also work.
Combining Query and Search Params
If you need both query and search parameters, you can set up type safety like this:
import { Text } from 'react-native';
import { useLocalSearchParams } from 'expo-router';
export default function Page() {
const { query, profile, search } = useLocalSearchParams<
'/[profile]/[...search]',
{ query?: string }
>();
return <Text>Search: {query ?? 'unset'}</Text>;
}
Conclusion
Adding type safety to your Expo Router setup can significantly enhance your development process by reducing bugs and improving code reliability. I highly recommend incorporating type safety into your applications to catch potential issues early and improve overall maintainability.
Try BanKan Board — The Project Management App Made for Developers, by Developers
If you’re tired of complicated project management tools with story points, sprints, and endless processes, BanKan Board is here to simplify your workflow. Built with developers in mind, BanKan Board lets you manage your projects without the clutter.
Key Features:
- No complicated processes: Focus on what matters without the overhead of traditional project management systems.
- Claude AI Assistant: Get smart assistance to streamline your tasks and improve productivity.
- Free to Use: Start using it without any upfront cost.
- Premium Features: Upgrade to unlock advanced functionality tailored to your team’s needs.
Whether you’re building a side project, managing a team, or collaborating on open-source software, BanKan Board is designed to make your life easier. Try it today!