Calendar UI Library for React Native
React Native offers numerous UI libraries, but finding a reliable calendar UI library can be challenging. Common issues include poor…
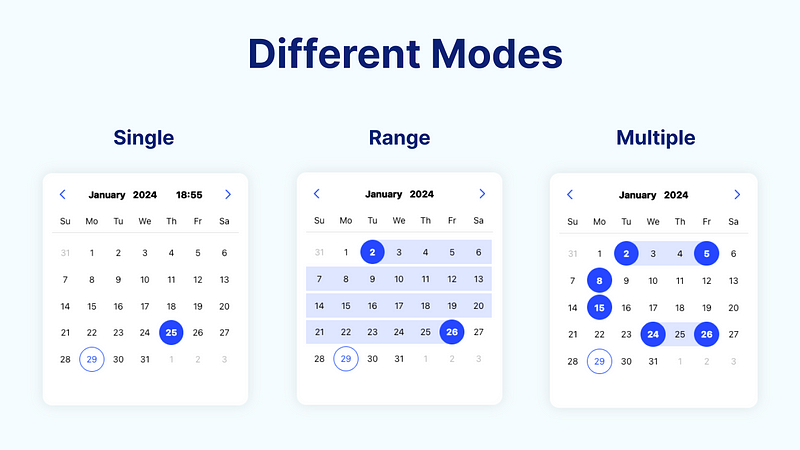
React Native offers numerous UI libraries, but finding a reliable calendar UI library can be challenging. Common issues include poor performance, slow animations, and rendering bugs. After extensive testing, I discovered react-native-ui-datepicker
, a TypeScript-based library that delivers outstanding performance and simplicity.
Although it lacks gesture support, its performance surpasses most alternatives, making it a compelling choice for many React Native projects.
Installation
- Install the Library:
yarn add react-native-ui-datepicker
2. Install Required Dependency:
yarn add dayjs
react-native-ui-datepicker
uses Day.js for date formatting. Day.js is a lightweight and fast alternative to Moment.js.
Basic Calendar Implementation
Below is a simple example of a calendar allowing single-day selection:
import React, { useState } from 'react';
import { View, StyleSheet } from 'react-native';
import DateTimePicker from 'react-native-ui-datepicker';
import dayjs from 'dayjs';
export default function App() {
const [date, setDate] = useState(dayjs());
return (
<View style={styles.container}>
<DateTimePicker
mode="single"
date={date}
onChange={(params) => setDate(params.date)}
/>
</View>
);
}
const styles = StyleSheet.create({
container: {
flex: 1,
backgroundColor: '#F5FCFF',
},
});
This basic example demonstrates how to allow users to select a single date.
Range Date Picker Example
To enable date range selection, configure the component in range
mode:
import React, { useState } from 'react';
import { View, StyleSheet } from 'react-native';
import DateTimePicker, { DateType } from 'react-native-ui-datepicker';
export default function App() {
const [range, setRange] = useState<{
startDate: DateType;
endDate: DateType;
}>({ startDate: undefined, endDate: undefined });
return (
<View style={styles.container}>
<DateTimePicker
mode="range"
startDate={range.startDate}
endDate={range.endDate}
onChange={(params) => setRange(params)}
/>
</View>
);
}
const styles = StyleSheet.create({
container: {
flex: 1,
backgroundColor: '#F5FCFF',
},
});
This configuration enables the user to select a start and end date for a range.
Multiple Date Picker Example
For selecting multiple dates, use the multiple
mode:
import React, { useState } from 'react';
import { View, StyleSheet } from 'react-native';
import DateTimePicker, { DateType } from 'react-native-ui-datepicker';
export default function App() {
const [dates, setDates] = useState<DateType[] | undefined>();
return (
<View style={styles.container}>
<DateTimePicker
mode="multiple"
dates={dates}
onChange={(params) => setDates(params.dates)}
/>
</View>
);
}
const styles = StyleSheet.create({
container: {
flex: 1,
backgroundColor: '#F5FCFF',
},
});
This mode allows users to select multiple non-contiguous dates.
Time Picker Support
In addition to date pickers, this library also supports time pickers, providing a versatile solution for date and time selection.
Cross-Platform Consistency
One of the standout features of react-native-ui-datepicker
is its consistent appearance across both iOS and Android platforms, ensuring a unified design for your mobile application.
Conclusion
react-native-ui-datepicker
is a robust and simple solution for implementing calendar UIs in React Native. Its performance, ease of use, and cross-platform consistency make it a strong contender for projects requiring date pickers. While it lacks gesture support, the trade-off in performance is worth considering.
Start using react-native-ui-datepicker
today to elevate your app’s calendar functionality!
Try BanKan Board — The Project Management App Made for Developers, by Developers
If you’re tired of complicated project management tools with story points, sprints, and endless processes, BanKan Board is here to simplify your workflow. Built with developers in mind, BanKan Board lets you manage your projects without the clutter.
Key Features:
- No complicated processes: Focus on what matters without the overhead of traditional project management systems.
- Claude AI Assistant: Get smart assistance to streamline your tasks and improve productivity.
- Free to Use: Start using it without any upfront cost.
- Premium Features: Upgrade to unlock advanced functionality tailored to your team’s needs.
Whether you’re building a side project, managing a team, or collaborating on open-source software, BanKan Board is designed to make your life easier. Try it today!